mirror of
https://github.com/Aider-AI/aider.git
synced 2025-05-25 23:05:00 +00:00
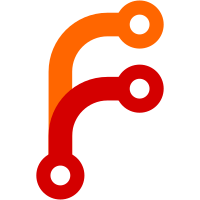
# Aider chat conversation: USER: ImportError: Failed to import test module: test_repomap Traceback (most recent call last): File "/opt/hostedtoolcache/Python/3.8.17/x64/lib/python3.8/unittest/loader.py", line 436, in _find_test_path module = self._get_module_from_name(name) File "/opt/hostedtoolcache/Python/3.8.17/x64/lib/python3.8/unittest/loader.py", line 377, in _get_module_from_name __import__(name) File "/home/runner/work/aider/aider/tests/test_repomap.py", line 8, in <module> from .utils import IgnorantTemporaryDirectory ImportError: attempted relative import with no known parent package ASSISTANT: The error message indicates that Python is unable to find the `utils` module when it's being imported in `test_repomap.py`. This is because the import statement is using a relative import with the dot (`.`) prefix, which is not working in this context. To fix this, we need to change the relative import to an absolute import. This means we need to specify the full path to the `utils` module from the project's root directory. Here is the change you need to make: ```python tests/test_repomap.py <<<<<<< ORIGINAL from .utils import IgnorantTemporaryDirectory ======= from tests.utils import IgnorantTemporaryDirectory >>>>>>> UPDATED ``` This change tells Python to import the `IgnorantTemporaryDirectory` class from the `utils` module located in the `tests` directory, which is an absolute path from the project's root directory.
148 lines
5.2 KiB
Python
148 lines
5.2 KiB
Python
import os
|
|
import unittest
|
|
from unittest.mock import patch
|
|
|
|
from aider.io import InputOutput
|
|
from aider.repomap import RepoMap
|
|
|
|
from tests.utils import IgnorantTemporaryDirectory
|
|
|
|
|
|
class TestRepoMap(unittest.TestCase):
|
|
def test_get_repo_map(self):
|
|
# Create a temporary directory with sample files for testing
|
|
test_files = [
|
|
"test_file1.py",
|
|
"test_file2.py",
|
|
"test_file3.md",
|
|
"test_file4.json",
|
|
]
|
|
|
|
with IgnorantTemporaryDirectory() as temp_dir:
|
|
for file in test_files:
|
|
with open(os.path.join(temp_dir, file), "w") as f:
|
|
f.write("")
|
|
|
|
io = InputOutput()
|
|
repo_map = RepoMap(root=temp_dir, io=io)
|
|
other_files = [os.path.join(temp_dir, file) for file in test_files]
|
|
result = repo_map.get_repo_map([], other_files)
|
|
|
|
# Check if the result contains the expected tags map
|
|
self.assertIn("test_file1.py", result)
|
|
self.assertIn("test_file2.py", result)
|
|
self.assertIn("test_file3.md", result)
|
|
self.assertIn("test_file4.json", result)
|
|
|
|
# close the open cache files, so Windows won't error
|
|
del repo_map
|
|
|
|
def test_get_repo_map_with_identifiers(self):
|
|
# Create a temporary directory with a sample Python file containing identifiers
|
|
test_file1 = "test_file_with_identifiers.py"
|
|
file_content1 = """\
|
|
class MyClass:
|
|
def my_method(self, arg1, arg2):
|
|
return arg1 + arg2
|
|
|
|
def my_function(arg1, arg2):
|
|
return arg1 * arg2
|
|
"""
|
|
|
|
test_file2 = "test_file_import.py"
|
|
file_content2 = """\
|
|
from test_file_with_identifiers import MyClass
|
|
|
|
obj = MyClass()
|
|
print(obj.my_method(1, 2))
|
|
print(my_function(3, 4))
|
|
"""
|
|
|
|
test_file3 = "test_file_pass.py"
|
|
file_content3 = "pass"
|
|
|
|
with IgnorantTemporaryDirectory() as temp_dir:
|
|
with open(os.path.join(temp_dir, test_file1), "w") as f:
|
|
f.write(file_content1)
|
|
|
|
with open(os.path.join(temp_dir, test_file2), "w") as f:
|
|
f.write(file_content2)
|
|
|
|
with open(os.path.join(temp_dir, test_file3), "w") as f:
|
|
f.write(file_content3)
|
|
|
|
io = InputOutput()
|
|
repo_map = RepoMap(root=temp_dir, io=io)
|
|
other_files = [
|
|
os.path.join(temp_dir, test_file1),
|
|
os.path.join(temp_dir, test_file2),
|
|
os.path.join(temp_dir, test_file3),
|
|
]
|
|
result = repo_map.get_repo_map([], other_files)
|
|
|
|
# Check if the result contains the expected tags map with identifiers
|
|
self.assertIn("test_file_with_identifiers.py", result)
|
|
self.assertIn("MyClass", result)
|
|
self.assertIn("my_method", result)
|
|
self.assertIn("my_function", result)
|
|
self.assertIn("test_file_pass.py", result)
|
|
|
|
# close the open cache files, so Windows won't error
|
|
del repo_map
|
|
|
|
def test_check_for_ctags_failure(self):
|
|
with patch("subprocess.run") as mock_run:
|
|
mock_run.side_effect = Exception("ctags not found")
|
|
repo_map = RepoMap(io=InputOutput())
|
|
self.assertFalse(repo_map.has_ctags)
|
|
|
|
def test_check_for_ctags_success(self):
|
|
with patch("subprocess.check_output") as mock_run:
|
|
mock_run.side_effect = [
|
|
(
|
|
b"Universal Ctags 0.0.0(f25b4bb7)\n Optional compiled features: +wildcards,"
|
|
b" +regex, +gnulib_fnmatch, +gnulib_regex, +iconv, +option-directory, +xpath,"
|
|
b" +json, +interactive, +yaml, +case-insensitive-filenames, +packcc,"
|
|
b" +optscript, +pcre2"
|
|
),
|
|
(
|
|
b'{"_type": "tag", "name": "status", "path": "aider/main.py", "pattern": "/^ '
|
|
b' status = main()$/", "kind": "variable"}'
|
|
),
|
|
]
|
|
repo_map = RepoMap(io=InputOutput())
|
|
self.assertTrue(repo_map.has_ctags)
|
|
|
|
def test_get_repo_map_without_ctags(self):
|
|
# Create a temporary directory with a sample Python file containing identifiers
|
|
test_files = [
|
|
"test_file_without_ctags.py",
|
|
"test_file1.txt",
|
|
"test_file2.md",
|
|
"test_file3.json",
|
|
"test_file4.html",
|
|
"test_file5.css",
|
|
"test_file6.js",
|
|
]
|
|
|
|
with IgnorantTemporaryDirectory() as temp_dir:
|
|
for file in test_files:
|
|
with open(os.path.join(temp_dir, file), "w") as f:
|
|
f.write("")
|
|
|
|
repo_map = RepoMap(root=temp_dir, io=InputOutput())
|
|
repo_map.has_ctags = False # force it off
|
|
|
|
other_files = [os.path.join(temp_dir, file) for file in test_files]
|
|
result = repo_map.get_repo_map([], other_files)
|
|
|
|
# Check if the result contains each specific file in the expected tags map without ctags
|
|
for file in test_files:
|
|
self.assertIn(file, result)
|
|
|
|
# close the open cache files, so Windows won't error
|
|
del repo_map
|
|
|
|
|
|
if __name__ == "__main__":
|
|
unittest.main()
|