mirror of
https://github.com/Aider-AI/aider.git
synced 2025-06-30 08:20:44 +00:00
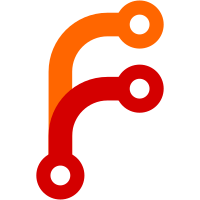
# Aider chat conversation: USER: the `scm_fname` is using a path in my local filesystem. how do i package up the queries directory for pip install in a way that the script can find those scm files at runtime ASSISTANT: To package the queries directory for pip install, you need to include it in your package data in the `setup.py` file. This will ensure that the directory is included when your package is installed. Then, you can use the `pkg_resources` module to access the files at runtime. This module is part of `setuptools`, so it's already installed if you're using pip. Here are the steps: 1. Modify `setup.py` to include the queries directory in the package data. ```python setup.py <<<<<<< HEAD packages=find_packages(), include_package_data=True, ======= packages=find_packages(), include_package_data=True, package_data={ '': ['queries/*'], }, >>>>>>> updated ``` 2. Modify `aider/repomap.py` to use `pkg_resources` to access the scm files at runtime. ```python aider/repomap.py <<<<<<< HEAD scm_fname = ( f"/Users/gauthier/tmp/py-tree-sitter-languages/queries/tree-sitter-{lang}-tags.scm" ) ======= scm_fname = pkg_resources.resource_filename( __name__, f"queries/tree-sitter-{lang}-tags.scm" ) >>>>>>> updated ``` Please note that the `queries` directory should be in the same directory as the `repomap.py` file for this to work. If it's in a different location, you'll need to adjust the path in the `resource_filename` call accordingly.
34 lines
939 B
Python
34 lines
939 B
Python
import re
|
|
|
|
from setuptools import find_packages, setup
|
|
|
|
with open("requirements.txt") as f:
|
|
requirements = f.read().splitlines()
|
|
|
|
from aider import __version__
|
|
|
|
with open("README.md", "r", encoding="utf-8") as f:
|
|
long_description = f.read()
|
|
long_description = re.sub(r"\n!\[.*\]\(.*\)", "", long_description)
|
|
long_description = re.sub(r"\n- \[.*\]\(.*\)", "", long_description)
|
|
|
|
setup(
|
|
name="aider-chat",
|
|
version=__version__,
|
|
packages=find_packages(),
|
|
include_package_data=True,
|
|
package_data={
|
|
'': ['queries/*'],
|
|
},
|
|
install_requires=requirements,
|
|
python_requires='>=3.9',
|
|
entry_points={
|
|
"console_scripts": [
|
|
"aider = aider.main:main",
|
|
],
|
|
},
|
|
description="aider is GPT powered coding in your terminal",
|
|
long_description=long_description,
|
|
long_description_content_type="text/markdown",
|
|
url="https://github.com/paul-gauthier/aider",
|
|
)
|