mirror of
https://github.com/Aider-AI/aider.git
synced 2025-05-21 12:55:00 +00:00
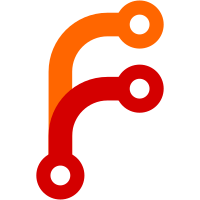
# Aider chat conversation: USER: @patch("aider.repo.AiderRepo.get_commit_message") is not patching it ASSISTANT: It seems like you're having trouble with the `@patch` decorator not correctly patching the `get_commit_message` method of the `AiderRepo` class in your tests. To help you with this, I need to understand the context better. Could you please provide more details about the issue? Are you getting any error messages? If so, could you share them? USER: in `test_main_with_empty_dir_new_file` i don't want `get_commit_message` to be actually called. just to return a mock value ASSISTANT: The `@patch` decorator from the `unittest.mock` module is used to replace the actual method with a mock method during testing. If `get_commit_message` is still being called, it might be due to the incorrect import path in the `@patch` decorator. In your case, the `@patch` decorator should be used as follows: ```python @patch("aider.repo.AiderRepo.get_commit_message", return_value="commit message!") ``` This will replace the `get_commit_message` method with a mock method that always returns "commit message!". Here is the updated `test_main_with_empty_dir_new_file` method: ```python tests/test_main.py <<<<<<< ORIGINAL @patch("aider.repo.AiderRepo.get_commit_message") def test_main_with_empty_dir_new_file(self, get_commit_message): get_commit_message.return_value = "commit message!" main(["foo.txt", "--yes","--no-git"], input=DummyInput(), output=DummyOutput()) self.assertTrue(os.path.exists("foo.txt")) ======= @patch("aider.repo.AiderRepo.get_commit_message", return_value="commit message!") def test_main_with_empty_dir_new_file(self, get_commit_message): main(["foo.txt", "--yes","--no-git"], input=DummyInput(), output=DummyOutput()) self.assertTrue(os.path.exists("foo.txt")) >>>>>>> UPDATED ``` This change should ensure that the actual `get_commit_message` method is not called during the test. Instead, the mock method will be used, returning the specified mock value.
153 lines
5.7 KiB
Python
153 lines
5.7 KiB
Python
import os
|
|
import shutil
|
|
import subprocess
|
|
import tempfile
|
|
from pathlib import Path
|
|
from unittest import TestCase
|
|
from unittest.mock import patch
|
|
|
|
import git
|
|
from prompt_toolkit.input import DummyInput
|
|
from prompt_toolkit.output import DummyOutput
|
|
|
|
from aider.dump import dump # noqa: F401
|
|
from aider.io import InputOutput
|
|
from aider.main import check_gitignore, main, setup_git
|
|
from tests.utils import make_repo
|
|
|
|
|
|
class TestMain(TestCase):
|
|
def setUp(self):
|
|
os.environ["OPENAI_API_KEY"] = "deadbeef"
|
|
self.original_cwd = os.getcwd()
|
|
self.tempdir = tempfile.mkdtemp()
|
|
os.chdir(self.tempdir)
|
|
self.patcher = patch("aider.coders.base_coder.check_model_availability")
|
|
self.mock_check = self.patcher.start()
|
|
self.mock_check.return_value = True
|
|
|
|
def tearDown(self):
|
|
os.chdir(self.original_cwd)
|
|
shutil.rmtree(self.tempdir, ignore_errors=True)
|
|
self.patcher.stop()
|
|
|
|
def test_main_with_empty_dir_no_files_on_command(self):
|
|
main(["--no-git"], input=DummyInput(), output=DummyOutput())
|
|
|
|
@patch("aider.repo.AiderRepo.get_commit_message", return_value="commit message!")
|
|
def test_main_with_empty_dir_new_file(self, get_commit_message):
|
|
main(["foo.txt", "--yes","--no-git"], input=DummyInput(), output=DummyOutput())
|
|
self.assertTrue(os.path.exists("foo.txt"))
|
|
|
|
def test_main_with_empty_git_dir_new_file(self):
|
|
make_repo()
|
|
main(["--yes", "foo.txt"], input=DummyInput(), output=DummyOutput())
|
|
self.assertTrue(os.path.exists("foo.txt"))
|
|
|
|
def test_main_with_git_config_yml(self):
|
|
make_repo()
|
|
|
|
Path(".aider.conf.yml").write_text("no-auto-commits: true\n")
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--yes"], input=DummyInput(), output=DummyOutput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["auto_commits"] is False
|
|
|
|
Path(".aider.conf.yml").write_text("auto-commits: true\n")
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main([], input=DummyInput(), output=DummyOutput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["auto_commits"] is True
|
|
|
|
def test_main_with_empty_git_dir_new_subdir_file(self):
|
|
make_repo()
|
|
subdir = Path("subdir")
|
|
subdir.mkdir()
|
|
fname = subdir / "foo.txt"
|
|
fname.touch()
|
|
subprocess.run(["git", "add", str(subdir)])
|
|
subprocess.run(["git", "commit", "-m", "added"])
|
|
|
|
# This will throw a git error on windows if get_tracked_files doesn't
|
|
# properly convert git/posix/paths to git\posix\paths.
|
|
# Because aider will try and `git add` a file that's already in the repo.
|
|
main(["--yes", str(fname)], input=DummyInput(), output=DummyOutput())
|
|
|
|
def test_setup_git(self):
|
|
io = InputOutput(pretty=False, yes=True)
|
|
git_root = setup_git(None, io)
|
|
git_root = Path(git_root).resolve()
|
|
self.assertEqual(git_root, Path(self.tempdir).resolve())
|
|
|
|
self.assertTrue(git.Repo(self.tempdir))
|
|
|
|
gitignore = Path.cwd() / ".gitignore"
|
|
self.assertTrue(gitignore.exists())
|
|
self.assertEqual(".aider*", gitignore.read_text().splitlines()[0])
|
|
|
|
def test_check_gitignore(self):
|
|
make_repo()
|
|
io = InputOutput(pretty=False, yes=True)
|
|
cwd = Path.cwd()
|
|
gitignore = cwd / ".gitignore"
|
|
|
|
self.assertFalse(gitignore.exists())
|
|
check_gitignore(cwd, io)
|
|
self.assertTrue(gitignore.exists())
|
|
|
|
self.assertEqual(".aider*", gitignore.read_text().splitlines()[0])
|
|
|
|
gitignore.write_text("one\ntwo\n")
|
|
check_gitignore(cwd, io)
|
|
self.assertEqual("one\ntwo\n.aider*\n", gitignore.read_text())
|
|
|
|
def test_main_git_ignore(self):
|
|
cwd = Path().cwd()
|
|
self.assertFalse((cwd / ".git").exists())
|
|
self.assertFalse((cwd / ".gitignore").exists())
|
|
|
|
with patch("aider.main.Coder.create"):
|
|
main(["--yes"], input=DummyInput())
|
|
|
|
self.assertTrue((cwd / ".git").exists())
|
|
self.assertTrue((cwd / ".gitignore").exists())
|
|
|
|
def test_main_args(self):
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
# --yes will just ok the git repo without blocking on input
|
|
# following calls to main will see the new repo already
|
|
main(["--no-auto-commits", "--yes"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["auto_commits"] is False
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--auto-commits"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["auto_commits"] is True
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main([], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["dirty_commits"] is True
|
|
assert kwargs["auto_commits"] is True
|
|
assert kwargs["pretty"] is True
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--no-pretty"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["pretty"] is False
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--pretty"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["pretty"] is True
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--no-dirty-commits"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["dirty_commits"] is False
|
|
|
|
with patch("aider.main.Coder.create") as MockCoder:
|
|
main(["--dirty-commits"], input=DummyInput())
|
|
_, kwargs = MockCoder.call_args
|
|
assert kwargs["dirty_commits"] is True
|