mirror of
https://github.com/Aider-AI/aider.git
synced 2025-05-21 21:04:59 +00:00
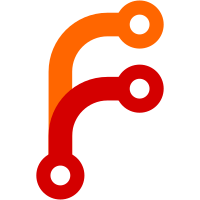
# Context: USER: I ran this command: pytest tests/test_commands.py Which produced this output: ============================= test session starts ============================== platform darwin -- Python 3.11.3, pytest-7.3.1, pluggy-1.0.0 rootdir: /Users/gauthier/Projects/aider collected 0 items / 1 error ==================================== ERRORS ==================================== ___________________ ERROR collecting tests/test_commands.py ____________________ ImportError while importing test module '/Users/gauthier/Projects/aider/tests/test_commands.py'. Hint: make sure your test modules/packages have valid Python names. Traceback: /usr/local/Cellar/python@3.11/3.11.3/Frameworks/Python.framework/Versions/3.11/lib/python3.11/importlib/__init__.py:126: in import_module return _bootstrap._gcd_import(name[level:], package, level) tests/test_commands.py:6: in <module> from aider.io import IO E ImportError: cannot import name 'IO' from 'aider.io' (/Users/gauthier/Projects/aider/aider/io.py) =========================== short test summary info ============================ ERROR tests/test_commands.py !!!!!!!!!!!!!!!!!!!! Interrupted: 1 error during collection !!!!!!!!!!!!!!!!!!!! =============================== 1 error in 0.33s =============================== ASSISTANT: It seems like there is an issue with importing the `IO` class from `aider.io`. Please provide the content of the `aider/io.py` file so I can help you resolve the issue. USER: Please note that I shared content of these additional files: aider/io.py ASSISTANT: Apologies for the oversight. I see the issue now. The class name in `aider/io.py` is `InputOutput`, not `IO`. Let's update the import statement in the `tests/test_commands.py` file. ```python tests/test_commands.py <<<<<<< ORIGINAL from aider.io import IO ======= from aider.io import InputOutput as IO >>>>>>> UPDATED ``` This change should fix the ImportError when running the tests.
27 lines
948 B
Python
27 lines
948 B
Python
import os
|
|
import tempfile
|
|
import unittest
|
|
from unittest.mock import MagicMock
|
|
from aider.commands import Commands
|
|
from aider.io import InputOutput as IO
|
|
from aider.coder import Coder
|
|
|
|
class TestCommands(unittest.TestCase):
|
|
def test_cmd_add(self):
|
|
with tempfile.TemporaryDirectory() as tmpdir:
|
|
io = IO()
|
|
coder = Coder(root=tmpdir)
|
|
commands = Commands(io, coder)
|
|
|
|
# Mock the Confirm.ask method to return True for creating files
|
|
with unittest.mock.patch("rich.prompt.Confirm.ask", return_value=True):
|
|
commands.cmd_add("foo.txt bar.txt")
|
|
|
|
foo_path = os.path.join(tmpdir, "foo.txt")
|
|
bar_path = os.path.join(tmpdir, "bar.txt")
|
|
|
|
self.assertTrue(os.path.exists(foo_path), "foo.txt should be created")
|
|
self.assertTrue(os.path.exists(bar_path), "bar.txt should be created")
|
|
|
|
if __name__ == "__main__":
|
|
unittest.main()
|