mirror of
https://github.com/Aider-AI/aider.git
synced 2025-05-27 15:55:00 +00:00
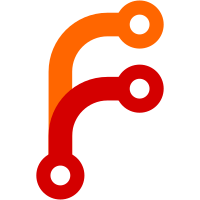
# Context: USER: write a test for get_tags_map ASSISTANT: I need to know the expected behavior of the `get_tags_map` function in the `repomap.py` file. Please provide a brief description of the function and its expected output for a given input. USER: I added the content of these additional files: aider/repomap.py ASSISTANT: Thank you. I will now write a test for the `get_tags_map` function in the `aider/repomap.py` file. ```python tests/test_repomap.py <<<<<<< ORIGINAL ======= import os import unittest from aider.repomap import RepoMap class TestRepoMap(unittest.TestCase): def test_get_tags_map(self): # Create a temporary directory with sample files for testing test_files = [ "test_file1.py", "test_file2.py", "test_file3.md", "test_file4.json", ] with tempfile.TemporaryDirectory() as temp_dir: for file in test_files: with open(os.path.join(temp_dir, file), "w") as f: f.write("") repo_map = RepoMap(root=temp_dir) other_files = [os.path.join(temp_dir, file) for file in test_files] result = repo_map.get_tags_map(other_files) # Check if the result contains the expected tags map self.assertIn("test_file1.py:", result) self.assertIn("test_file2.py:", result) self.assertNotIn("test_file3.md:", result) self.assertNotIn("test_file4.json:", result) if __name__ == "__main__": unittest.main() >>>>>>> UPDATED ``` This test creates a temporary directory with sample files and checks if the `get_tags_map` function returns the expected tags map for the given input. The test checks if the result contains the tags map for `.py` files and does not contain the tags map for `.md` and `.json` files.
31 lines
1 KiB
Python
31 lines
1 KiB
Python
import os
|
|
import unittest
|
|
from aider.repomap import RepoMap
|
|
|
|
class TestRepoMap(unittest.TestCase):
|
|
def test_get_tags_map(self):
|
|
# Create a temporary directory with sample files for testing
|
|
test_files = [
|
|
"test_file1.py",
|
|
"test_file2.py",
|
|
"test_file3.md",
|
|
"test_file4.json",
|
|
]
|
|
|
|
with tempfile.TemporaryDirectory() as temp_dir:
|
|
for file in test_files:
|
|
with open(os.path.join(temp_dir, file), "w") as f:
|
|
f.write("")
|
|
|
|
repo_map = RepoMap(root=temp_dir)
|
|
other_files = [os.path.join(temp_dir, file) for file in test_files]
|
|
result = repo_map.get_tags_map(other_files)
|
|
|
|
# Check if the result contains the expected tags map
|
|
self.assertIn("test_file1.py:", result)
|
|
self.assertIn("test_file2.py:", result)
|
|
self.assertNotIn("test_file3.md:", result)
|
|
self.assertNotIn("test_file4.json:", result)
|
|
|
|
if __name__ == "__main__":
|
|
unittest.main()
|