mirror of
https://github.com/Aider-AI/aider.git
synced 2025-05-20 04:14:59 +00:00
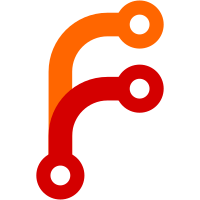
# Aider chat conversation: USER: why is it thinking that 0.9.0 is newer than 0.9.0-dev ASSISTANT: The version comparison is being done using the `packaging.version.parse` function. This function follows the PEP 440 version identification and comparison rules. According to PEP 440, a version like "0.9.0-dev" is considered a pre-release of "0.9.0", and therefore "0.9.0" is considered newer. If you want "0.9.0-dev" to be considered newer than "0.9.0", you would need to change the version comparison logic. However, this would be against the standard Python versioning rules and is not recommended. If you still want to proceed with this, please confirm and I will provide the necessary changes. USER: I added these *read-write* files: scripts/versionbump.py ASSISTANT: I understand that you want to modify the version comparison logic in your scripts. However, I would like to clarify where exactly you want this change to be made. The file `scripts/versionbump.py` does not seem to have any version comparison logic that would consider "0.9.0 ^C KeyboardInterrupt USER: instead of adding -dev into the version after the bump, can you instead increment the version by 0.0.1 and then add dev ASSISTANT: Sure, we can modify the `scripts/versionbump.py` script to increment the version by 0.0.1 and then add "-dev" to it. Here's how we can do it: 1. After the new version is parsed, we can increment the patch version by 1. 2. Then, we can add "-dev" to this incremented version. Here's the change: ```python scripts/versionbump.py <<<<<<< ORIGINAL new_version = version.parse(new_version_str) with open("aider/__init__.py", "r") as f: content = f.read() ======= new_version = version.parse(new_version_str) incremented_version = version.Version(f"{new_version.major}.{new_version.minor}.{new_version.micro + 1}") with open("aider/__init__.py", "r") as f: content = f.read() >>>>>>> UPDATED ``` ```python scripts/versionbump.py <<<<<<< ORIGINAL updated_dev_content = re.sub( r'__version__ = ".+?"', f'__version__ = "{new_version}-dev"', content ) ======= updated_dev_content = re.sub( r'__version__ = ".+?"', f'__version__ = "{incremented_version}-dev"', content ) >>>>>>> UPDATED ``` This will increment the patch version by 0.0.1 and then add "-dev" to it after the version bump.
78 lines
2.5 KiB
Python
78 lines
2.5 KiB
Python
import argparse
|
|
import re
|
|
import subprocess
|
|
|
|
from packaging import version
|
|
|
|
|
|
def main():
|
|
parser = argparse.ArgumentParser(description="Bump version")
|
|
parser.add_argument("new_version", help="New version in x.y.z format")
|
|
parser.add_argument(
|
|
"--dry-run", action="store_true", help="Print each step without actually executing them"
|
|
)
|
|
args = parser.parse_args()
|
|
dry_run = args.dry_run
|
|
|
|
new_version_str = args.new_version
|
|
if not re.match(r"^\d+\.\d+\.\d+$", new_version_str):
|
|
raise ValueError(f"Invalid version format, must be x.y.z: {new_version_str}")
|
|
|
|
new_version = version.parse(new_version_str)
|
|
incremented_version = version.Version(f"{new_version.major}.{new_version.minor}.{new_version.micro + 1}")
|
|
|
|
with open("aider/__init__.py", "r") as f:
|
|
content = f.read()
|
|
|
|
current_version = re.search(r'__version__ = "(.+?)"', content).group(1).split("-dev")[0]
|
|
if new_version <= version.parse(current_version):
|
|
raise ValueError(
|
|
f"New version {new_version} must be greater than the current version {current_version}"
|
|
)
|
|
|
|
updated_content = re.sub(r'__version__ = ".+?"', f'__version__ = "{new_version}"', content)
|
|
|
|
print("Updating aider/__init__.py with new version:")
|
|
print(updated_content)
|
|
if not dry_run:
|
|
with open("aider/__init__.py", "w") as f:
|
|
f.write(updated_content)
|
|
|
|
git_commands = [
|
|
["git", "add", "aider/__init__.py"],
|
|
["git", "commit", "-m", f"version bump to {new_version}"],
|
|
["git", "tag", f"v{new_version}"],
|
|
["git", "push", "origin"],
|
|
["git", "push", "origin", f"v{new_version}"],
|
|
]
|
|
|
|
for cmd in git_commands:
|
|
print(f"Running: {' '.join(cmd)}")
|
|
if not dry_run:
|
|
subprocess.run(cmd, check=True)
|
|
|
|
updated_dev_content = re.sub(
|
|
r'__version__ = ".+?"', f'__version__ = "{incremented_version}-dev"', content
|
|
)
|
|
|
|
print()
|
|
print("Updating aider/__init__.py with new dev version:")
|
|
print(updated_dev_content)
|
|
if not dry_run:
|
|
with open("aider/__init__.py", "w") as f:
|
|
f.write(updated_dev_content)
|
|
|
|
git_commands_dev = [
|
|
["git", "add", "aider/__init__.py"],
|
|
["git", "commit", "-m", f"set version to {new_version}-dev"],
|
|
["git", "push", "origin"],
|
|
]
|
|
|
|
for cmd in git_commands_dev:
|
|
print(f"Running: {' '.join(cmd)}")
|
|
if not dry_run:
|
|
subprocess.run(cmd, check=True)
|
|
|
|
|
|
if __name__ == "__main__":
|
|
main()
|