mirror of
https://github.com/mudler/LocalAI.git
synced 2025-05-20 18:45:00 +00:00
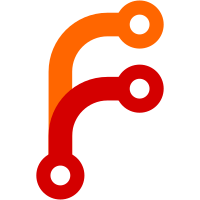
fix(model-list): be consistent, skip known files from listing This changeset does two things: - Removes the dependency of listing models from the OpenAI schema. - Tries to reduce confusion between ListModels() in model loader and in the service - now there is only one ListModels which is in services and does not depend anymore on the OpenAI schema - The OpenAI-schema functions were moved nearby the OpenAI specific endpoints that needs the schema - Drops the ListModel Service structure as there was no real need for it. Signed-off-by: Ettore Di Giacinto <mudler@localai.io>
57 lines
1.3 KiB
Go
57 lines
1.3 KiB
Go
package services
|
|
|
|
import (
|
|
"regexp"
|
|
|
|
"github.com/mudler/LocalAI/core/config"
|
|
"github.com/mudler/LocalAI/pkg/model"
|
|
)
|
|
|
|
func ListModels(bcl *config.BackendConfigLoader, ml *model.ModelLoader, filter string, excludeConfigured bool) ([]string, error) {
|
|
|
|
models, err := ml.ListFilesInModelPath()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var mm map[string]interface{} = map[string]interface{}{}
|
|
|
|
dataModels := []string{}
|
|
|
|
var filterFn func(name string) bool
|
|
|
|
// If filter is not specified, do not filter the list by model name
|
|
if filter == "" {
|
|
filterFn = func(_ string) bool { return true }
|
|
} else {
|
|
// If filter _IS_ specified, we compile it to a regex which is used to create the filterFn
|
|
rxp, err := regexp.Compile(filter)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
filterFn = func(name string) bool {
|
|
return rxp.MatchString(name)
|
|
}
|
|
}
|
|
|
|
// Start with the known configurations
|
|
for _, c := range bcl.GetAllBackendConfigs() {
|
|
if excludeConfigured {
|
|
mm[c.Model] = nil
|
|
}
|
|
|
|
if filterFn(c.Name) {
|
|
dataModels = append(dataModels, c.Name)
|
|
}
|
|
}
|
|
|
|
// Then iterate through the loose files:
|
|
for _, m := range models {
|
|
// And only adds them if they shouldn't be skipped.
|
|
if _, exists := mm[m]; !exists && filterFn(m) {
|
|
dataModels = append(dataModels, m)
|
|
}
|
|
}
|
|
|
|
return dataModels, nil
|
|
}
|